
How can I retrieve the HTML source of a specific WebElement in Selenium WebDriver using Python?
I am using Python with Selenium WebDriver and can perform basic operations like locating elements and fetching the full page source.
To retrieve the HTML source of a specific WebElement (including its children) in Selenium WebDriver using Python, you can use the get_attribute('outerHTML') method. Here's how you can achieve it:
Solution
from selenium import webdriver
# Set up the WebDriver
driver = webdriver.Firefox() # You can use any browser WebDriver
driver.get("https://example.com") # Replace with your target URL
# Locate the element
element = driver.find_element_by_css_selector("#my-id") # Replace with your desired CSS selector
# Get the HTML source of the element
element_html = element.get_attribute("outerHTML")
print(element_html)
# Close the browser
driver.quit()
Explanation
Locate the Element:
Use methods like find_element_by_css_selector, find_element_by_id, or find_element (with By locators) to locate the desired WebElement.
Retrieve HTML Source:
outerHTML: Returns the HTML of the element including the element itself and all its children.
innerHTML: Returns the HTML inside the element, excluding the element itself.
Example:
If the HTML is:
Hello World
outerHTML will return:
Hello World
innerHTML will return:
Hello World
Print or Process the HTML:
The get_attribute("outerHTML") method returns the HTML as a string, which you can print or further process in your script.
Notes
- Replace #my-id with the actual CSS selector or XPath of the element you want to target.
If you're using Selenium 4, prefer find_element with By locators:
from selenium.webdriver.common.by import By
element = driver.find_element(By.CSS_SELECTOR, "#my-id")
Your Answer
Answers (23)
Wow, this post is amazing! Your insights on travel hacks are so practical and helpful. I’ve been looking for ways to make my trips more enjoyable, and your tips are spot on. I’ll definitely try out the packing tricks you mentioned. Thanks for sharing such valuable information.
good information
1 Month
Wow, What a Excellent post. I really found this to much informatics. It is what i was searching for.I would like to suggest you that please keep sharing such type of info.Thanks
1 Month
I really appreciate the balanced approach you’ve taken in this blog post. It’s evident that you’ve considered multiple viewpoints before forming your conclusions. Instead of presenting a one-sided argument, you’ve created a well-rounded discussion that allows readers to form their own opinions. This kind of fair, thoughtful writing is what makes your blog so valuable. It’s clear that you prioritize quality and integrity in your content, and that’s something that truly stands out. Keep up the great work!
get more info
1 Month
it is a really nice point of view. I usually meet people who rather say what they suppose others want to hear. Good and well written! I will come back to your site for sure!I was reading through some of your blog posts on this website and I conceive this website is rattling instructive! Retain posting.
Find out
1 Month
I am happy to find this post very useful for me, as it contains lot of information. I always prefer to read the quality content and this thing I found in you post. Thanks for sharing. I also wanna talk about the
View data
1 Month
I am happy to find this post very useful for me, as it contains lot of information. I always prefer to read the quality content and this thing I found in you post. Thanks for sharing. I also wanna talk about the
brothertoto
1 Month
There is some great power that has been discovered using green coffee weight loss especially in America. With a lot of misinformation in this modern world, it is becoming more essential for people to take responsibility of their health through getting the right information. Weight loss is no different, it to has been crowded with people claiming they can make you lose instantly but all in the wrong ways.
Learn more
1 Month
There a few interesting points soon enough on this page but I do not determine if they all center to heart. There is some validity but I will take hold opinion until I check into it further. Great post , thanks and that we want a lot more! Put into FeedBurner at the same time
click here
1 Month
While looking for articles on these topics, I came across this article on the site here. As I read your article, I felt like an expert in this field. I have several articles on these topics posted on my site. Could you please visit my homepage?
good information
1 Month
Your website is a true example of how great content should be delivered. The design is user-friendly, and every detail feels well-thought-out. I admire how your posts are filled with valuable information yet remain easy to understand. It’s always a pleasure to learn something new here. Your dedication to sharing quality content inspires me to think about how I can better engage my audience. Please keep sharing your amazing insights because they’re a big help to readers like me. Thank you for all your hard work and creativity!
website
1 Month
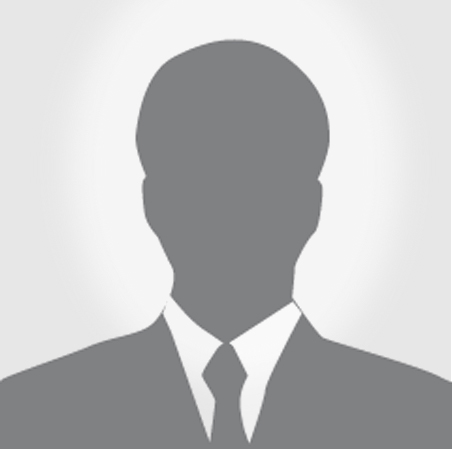

Wow, this post is amazing! Your insights on travel hacks are so practical and helpful. I’ve been looking for ways to make my trips more enjoyable, and your tips are spot on. I’ll definitely try out the packing tricks you mentioned. Thanks for sharing such valuable information.
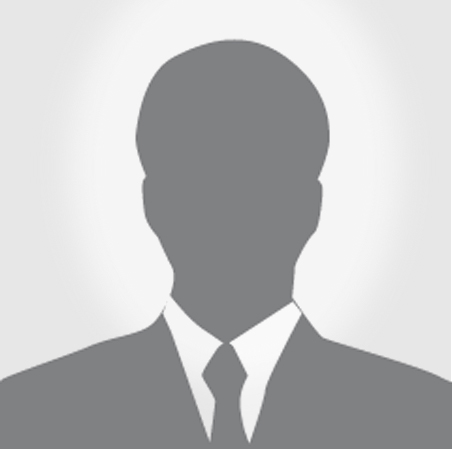

Wow, What a Excellent post. I really found this to much informatics. It is what i was searching for.I would like to suggest you that please keep sharing such type of info.Thanks
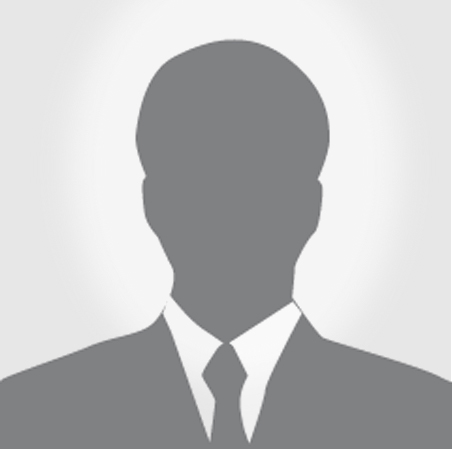

I really appreciate the balanced approach you’ve taken in this blog post. It’s evident that you’ve considered multiple viewpoints before forming your conclusions. Instead of presenting a one-sided argument, you’ve created a well-rounded discussion that allows readers to form their own opinions. This kind of fair, thoughtful writing is what makes your blog so valuable. It’s clear that you prioritize quality and integrity in your content, and that’s something that truly stands out. Keep up the great work!
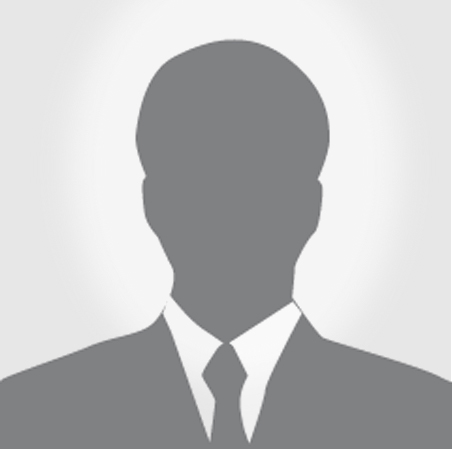

it is a really nice point of view. I usually meet people who rather say what they suppose others want to hear. Good and well written! I will come back to your site for sure!I was reading through some of your blog posts on this website and I conceive this website is rattling instructive! Retain posting.
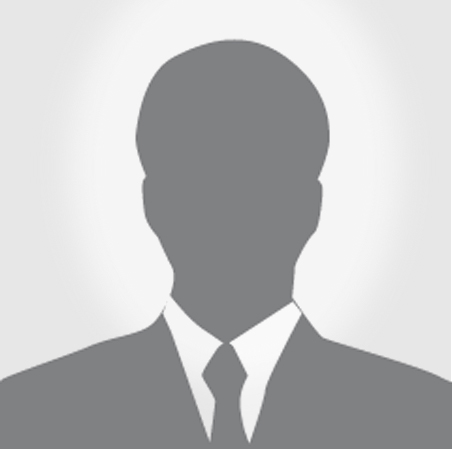

I am happy to find this post very useful for me, as it contains lot of information. I always prefer to read the quality content and this thing I found in you post. Thanks for sharing. I also wanna talk about the
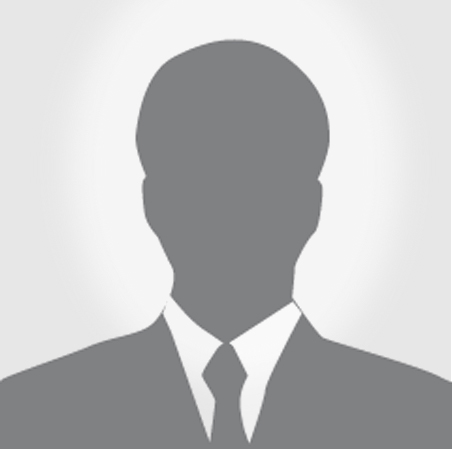

I am happy to find this post very useful for me, as it contains lot of information. I always prefer to read the quality content and this thing I found in you post. Thanks for sharing. I also wanna talk about the
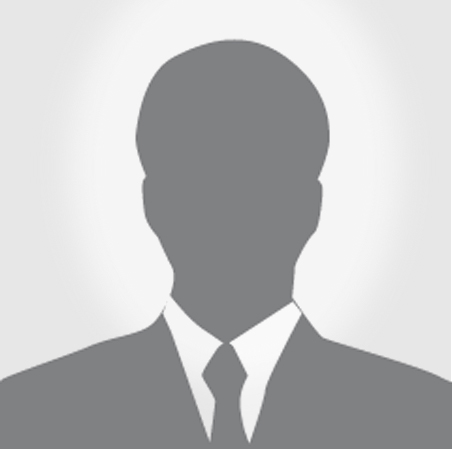

There is some great power that has been discovered using green coffee weight loss especially in America. With a lot of misinformation in this modern world, it is becoming more essential for people to take responsibility of their health through getting the right information. Weight loss is no different, it to has been crowded with people claiming they can make you lose instantly but all in the wrong ways.
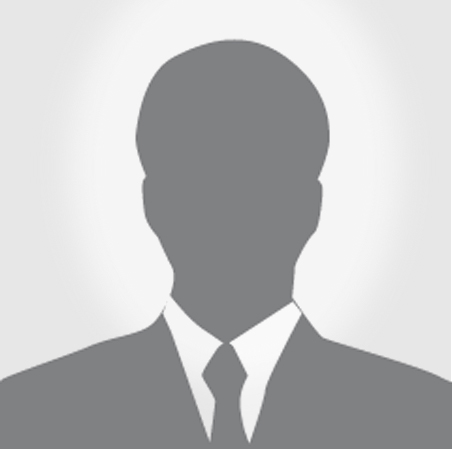

There a few interesting points soon enough on this page but I do not determine if they all center to heart. There is some validity but I will take hold opinion until I check into it further. Great post , thanks and that we want a lot more! Put into FeedBurner at the same time
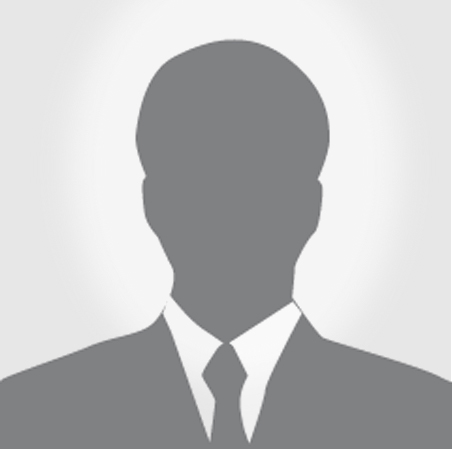

While looking for articles on these topics, I came across this article on the site here. As I read your article, I felt like an expert in this field. I have several articles on these topics posted on my site. Could you please visit my homepage?
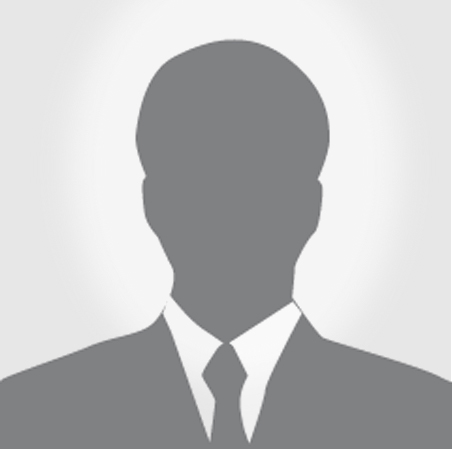

Your website is a true example of how great content should be delivered. The design is user-friendly, and every detail feels well-thought-out. I admire how your posts are filled with valuable information yet remain easy to understand. It’s always a pleasure to learn something new here. Your dedication to sharing quality content inspires me to think about how I can better engage my audience. Please keep sharing your amazing insights because they’re a big help to readers like me. Thank you for all your hard work and creativity!
website