How to locate a and verify if the account update was successful?
I have been tasked with writing automated test scripts by using the Selenium WebDriver to verify the presence and content of a element on a webpage. How can I achieve the following Target:-
Locating the element by its I’d.Is the displayed on the page?Verify that the text within the matches “your account has been successfully updated”
you can accomplish this particular task by using the selenium WebDriver in Python programming language by using the following coding snippet:-
- From selenium import webdriver
- From selenium.webdriver.common.by import By
- From selenium.webdriver.support.ui import WebDriverWait
- From selenium.webdriver.support import expected_conditions as EC
# Initialize the WebDriver (assuming Chrome here)
Driver = webdriver.Chrome()
# Open the website
Driver.get(https://www.example.com)
Try:
# Locate the element by its ID
Div_element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, “div_id”))
)
# Check if the is displayed on the page
If div_element.is_displayed():
# Verify the text within the
Div_text = div_element.text
If div_text == “your account has been successfully updated”:
Print(“The text within the matches the expected text.”)
Else:
Print(“The text within the does not match the expected text.”)
Else:
Print(“The element is not displayed on the page.”)
Except Exception as e:
# Handle any exceptions that may occur during the process
Print(f”An error occurred: {e}”)
Finally:
# Close the WebDriver
Driver.quit()
Here is how you can achieve this task by using the java programming language:-
Import org.openqa.selenium.By;
Import org.openqa.selenium.WebDriver;
Import org.openqa.selenium.WebElement;
Import org.openqa.selenium.chrome.ChromeDriver;
Import org.openqa.selenium.support.ui.ExpectedConditions;
Import org.openqa.selenium.support.ui.WebDriverWait;
Public class VerifyDivText {
Public static void main(String[] args) {
// Set the path to the ChromeDriver executable
System.setProperty(“webdriver.chrome.driver”, “/path/to/chromedriver”);
// Initialize the ChromeDriver
WebDriver driver = new ChromeDriver();
// Open the website
Driver.get(https://www.example.com);
Try {
// Locate the element by its ID
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement divElement = wait.until(ExpectedConditions.presenceOfElementLocated(By.id(“div_id”)));
// Check if the is displayed on the page
If (divElement.isDisplayed()) {
// Verify the text within the
String divText = divElement.getText();
If (“your account has been successfully updated”.equals(divText)) {
System.out.println(“The text within the matches the expected text.”);
} else {
System.out.println(“The text within the does not match the expected text.”);
}
} else {
System.out.println(“The element is not displayed on the page.”);
}
} catch (Exception e) {
// Handle any exceptions that may occur during the process
System.err.println(“An error occurred: “ + e.getMessage());
} finally {
// Close the WebDriver
Driver.quit();
}
}
}
Here is how you can achieve this task by using the HTML:-
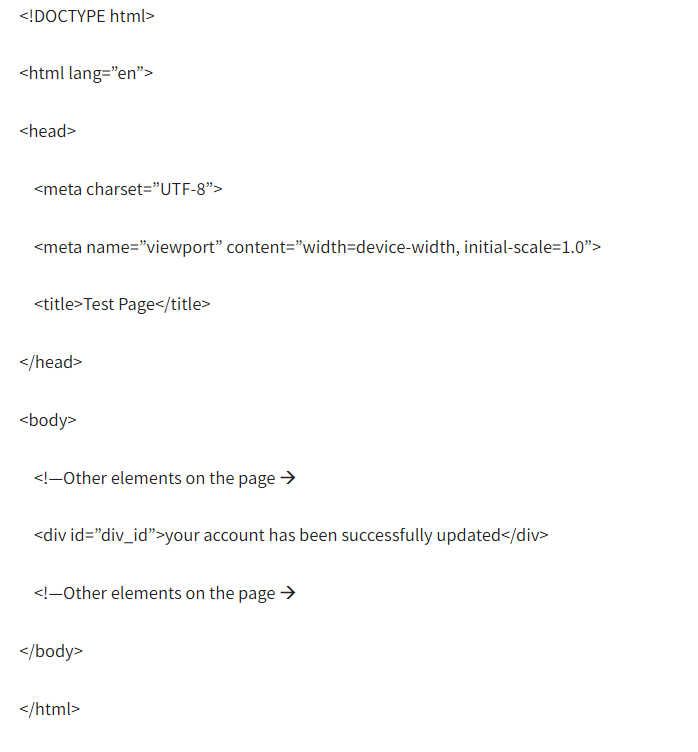
I have been tasked with writing automated test scripts by using the Selenium WebDriver to verify the presence and content of a Locating the Is the Verify that the text within the you can accomplish this particular task by using the selenium WebDriver in Python programming language by using the following coding snippet:- Here is how you can achieve this task by using the HTML:-
# Initialize the WebDriver (assuming Chrome here)
Driver = webdriver.Chrome()
# Open the website
Driver.get(https://www.example.com)
Try:
# Locate the element by its ID
Div_element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, “div_id”))
)
# Check if the is displayed on the page
If div_element.is_displayed():
# Verify the text within the
Div_text = div_element.text
If div_text == “your account has been successfully updated”:
Print(“The text within the matches the expected text.”)
Else:
Print(“The text within the does not match the expected text.”)
Else:
Print(“The element is not displayed on the page.”)
Except Exception as e:
# Handle any exceptions that may occur during the process
Print(f”An error occurred: {e}”)
Finally:
# Close the WebDriver
Driver.quit()
Here is how you can achieve this task by using the java programming language:-
Import org.openqa.selenium.By;
Import org.openqa.selenium.WebDriver;
Import org.openqa.selenium.WebElement;
Import org.openqa.selenium.chrome.ChromeDriver;
Import org.openqa.selenium.support.ui.ExpectedConditions;
Import org.openqa.selenium.support.ui.WebDriverWait;
Public class VerifyDivText {
Public static void main(String[] args) {
// Set the path to the ChromeDriver executable
System.setProperty(“webdriver.chrome.driver”, “/path/to/chromedriver”);
// Initialize the ChromeDriver
WebDriver driver = new ChromeDriver();
// Open the website
Driver.get(https://www.example.com);
Try {
// Locate the element by its ID
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement divElement = wait.until(ExpectedConditions.presenceOfElementLocated(By.id(“div_id”)));
// Check if the is displayed on the page
If (divElement.isDisplayed()) {
// Verify the text within the
String divText = divElement.getText();
If (“your account has been successfully updated”.equals(divText)) {
System.out.println(“The text within the matches the expected text.”);
} else {
System.out.println(“The text within the does not match the expected text.”);
}
} else {
System.out.println(“The element is not displayed on the page.”);
}
} catch (Exception e) {
// Handle any exceptions that may occur during the process
System.err.println(“An error occurred: “ + e.getMessage());
} finally {
// Close the WebDriver
Driver.quit();
}
}
}
Interviews
- Business Analyst Interview Questions
- DevOps Interview Questions
- AWS Interview Questions
- QA Testing Interview Questions
- Software Testing Interview Questions
- SQL Interview Questions
- Salesforce Interview Questions
- Java Interview Questions
- Hibernate Interview Questions
- Spark Interview Questions
- Vmware Interview Questions
- Data Science Interview Questions
- Digital Marketing Interview Questions
- API Testing Interview Questions
- SSAS Interview Questions
- Power BI Interview Questions
- Cloud Computing Interview Questions
- SSRS Interview Questions
- Manual Testing Interview Questions
- Social Media Interview Questions
- Performance Testing Interview Questions
- MSBI Interview Questions
- QTP Interview Questions
- Automation Testing Interview Questions
- SSIS Interview Questions
- GIT Interview Questions