To quickly get today's date in a Lightning Component and use it in an attribute, you can leverage JavaScript within your component's controller or helper. Here’s a step-by-step guide on how to achieve this:
Step 1: Define the Attribute in the Component
First, define an attribute in your Lightning Component to hold today's date.
Step 2: Set the Date in the Component's Controller or Helper
You need to set the value of the todayDate attribute when the component is initialized. You can do this in the controller or helper.
Option 1: Using Controller (JavaScript)
Create a controller file if it doesn’t already exist, and set the date when the component initializes.
({ doInit: function(component, event, helper) { // Get today's date let today = new Date(); // Format the date as a string (e.g., YYYY-MM-DD) let formattedDate = today.toISOString().split('T')[0]; // Set the attribute component.set("v.todayDate", formattedDate); }})Option 2: Using Helper (JavaScript)Alternatively, you can set the date in the helper and call it from the controller.javascriptCopy code// Controller({ doInit: function(component, event, helper) { helper.setTodayDate(component); }})// Helper({ setTodayDate: function(component) { // Get today's date let today = new Date(); // Format the date as a string (e.g., YYYY-MM-DD) let formattedDate = today.toISOString().split('T')[0]; // Set the attribute component.set("v.todayDate", formattedDate); }})
Step 3: Initialize the Component
Ensure that the doInit method is called when the component is initialized.
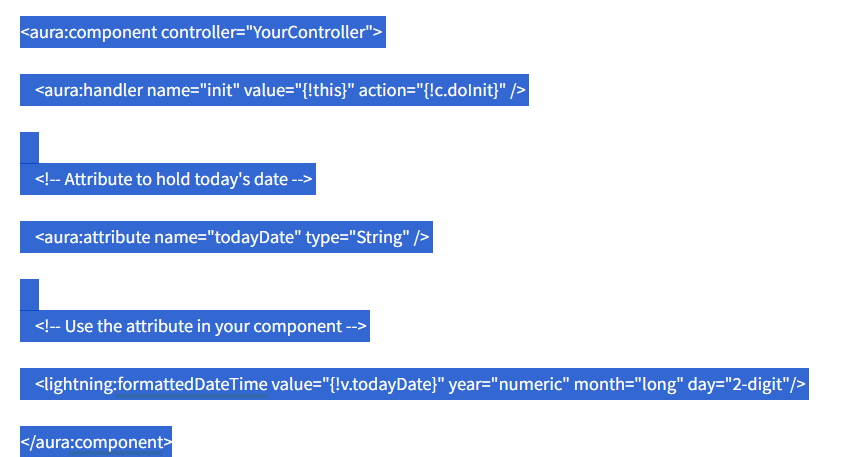
Explanation
Attribute Definition: The aura:attribute tag defines a component attribute named todayDate to store the date.
Initialization Handler: The aura:handler is used to call the doInit function when the component is initialized.
Controller or Helper Logic: The logic in the controller or helper gets today's date using JavaScript's Date object, formats it to a string (e.g., YYYY-MM-DD), and sets the component attribute todayDate.
This setup ensures that when your Lightning Component is initialized, it automatically gets and displays today's date.