
How to get the profile name and role name from User object
We already have a soql query to retrieve the User object (which returns the role id and profile id) was wondering if we could retrieve the RoleName and ProfileName from within the same query instead of making an additional query to the userInfo object. It will be of great help if you could please point us in the right direction
Note: Also we want to retrieve this for a seq of users so it would save a lot of additional calls if we could retrieve those details in the same call for the User object. How to get the profile name?
You can retrieve profile name, role name this way:
select id, name, , profile.name, userrole.name from user
For details on how this works, you can refer to the Name object's documentation.
Your Answer
Answers (3)
If you need to get the Profile Name and Role Name from the User object in Salesforce using SOQL or Apex, here’s how you can do it:
1. Using SOQL Query (Best Approach)
The User object has lookup relationships with both Profile and UserRole, so you need to query them like this:
SELECT Id, Name, Profile.Name, UserRole.Name FROM User WHERE Id = :UserInfo.getUserId()
Profile.Name → Fetches the profile name
UserRole.Name → Fetches the role name (can be null if no role is assigned)
2. Using Apex to Get Profile and Role Name
If you're working inside an Apex class, you can store these values like this:
User currentUser = [SELECT Id, Name, Profile.Name, UserRole.Name FROM User WHERE Id = :UserInfo.getUserId()];
System.debug('Profile Name: ' + currentUser.Profile.Name);
System.debug('Role Name: ' + currentUser.UserRole.Name);
UserInfo.getUserId() gets the current logged-in user’s ID.
The query retrieves Profile Name and User Role Name for that user.
3. Important Notes
Every user must have a Profile, but UserRole can be null.
If you only need the profile name, use:
System.debug(UserInfo.getProfileId());
However, this returns the Profile ID, not the name, so a query is needed.
4 Months
Use the SELECT clause in combination with Relationship Queries in SOQL to retrieve the fall guys names of Roles (UserRole.Name) and Profiles (Profile.Name) directly from the User table.SELECT Id, UserRoleId, ProfileId, UserRole.Name, Profile.NameSELECT Id, UserRoleId, ProfileId, UserRole.Name, Profile.Name
SELECT Id, UserRoleId, ProfileId, UserRole.Name, Profile.Name
FROM User
WHERE Id = 'USER_ID_HERE'
5 Months
To retrieve the profile name and role name from a user object, you typically need to access specific fields related to the user in your database or application. The exact method depends on the context (e.g., a Salesforce environment, a custom application with a database, etc.). Below, I'll provide examples for both Salesforce and a generic database application.
In Salesforce
In Salesforce, each user is associated with a profile and a role. You can retrieve the profile name and role name using SOQL (Salesforce Object Query Language).
Example SOQL Query
SELECT Id, Name, Profile.Name, UserRole.Name FROM User WHERE Id = 'someUserId'
Explanation
- Id: The unique identifier for the user.
- Name: The name of the user.
- Profile.Name: The name of the profile associated with the user.
- UserRole.Name: The name of the role associated with the user.
In a Custom Application with a Database
If you have a custom application with a relational database, you typically have tables like users, profiles, and roles. You can use SQL to join these tables and get the desired information.
Example SQL Query
SELECT users.id, users.name, profiles.name AS profile_name, roles.name AS role_name
FROM users
JOIN profiles ON users.profile_id = profiles.id
JOIN roles ON users.role_id = roles.id
WHERE users.id = 'someUserId';
- Explanation
- users.id: The unique identifier for the user.
- users.name: The name of the user.
- profiles.name: The name of the profile associated with the user.
- roles.name: The name of the role associated with the user.
- In a Programming Context
If you're working within a specific programming context (e.g., a Python application using an ORM), the approach will vary. Here’s an example using Python with SQLAlchemy:
Python with SQLAlchemy Example
from sqlalchemy.orm import sessionmaker
from your_model_definitions import User, Profile, Role
# Assuming you have a configured engine
Session = sessionmaker(bind=engine)
session = Session()
user_id = 'someUserId'
user = session.query(User).filter(User.id == user_id).first()
if user:
profile_name = user.profile.name
role_name = user.role.name
print(f"Profile Name: {profile_name}, Role Name: {role_name}")
else:
print("User not found")
Explanation
This code snippet uses SQLAlchemy to query a user and get the related profile and role names.
User, Profile, and Role are ORM models.
The relationships between User, Profile, and Role should be properly defined in your ORM models.
Conclusion
The exact method to retrieve the profile name and role name from a user object depends on the environment and tools you're using. Whether it's Salesforce with SOQL, a relational database with SQL, or an ORM in a programming language, the general approach involves querying the user and their associated profile and role.
1 Year
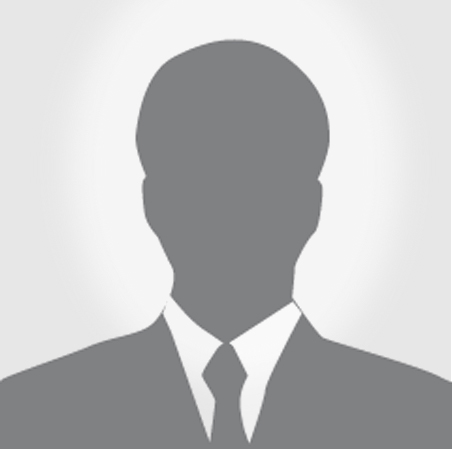

If you need to get the Profile Name and Role Name from the User object in Salesforce using SOQL or Apex, here’s how you can do it:
1. Using SOQL Query (Best Approach)
The User object has lookup relationships with both Profile and UserRole, so you need to query them like this:
SELECT Id, Name, Profile.Name, UserRole.Name FROM User WHERE Id = :UserInfo.getUserId()
Profile.Name → Fetches the profile name
UserRole.Name → Fetches the role name (can be null if no role is assigned)
2. Using Apex to Get Profile and Role Name
If you're working inside an Apex class, you can store these values like this:
User currentUser = [SELECT Id, Name, Profile.Name, UserRole.Name FROM User WHERE Id = :UserInfo.getUserId()];
System.debug('Profile Name: ' + currentUser.Profile.Name);
System.debug('Role Name: ' + currentUser.UserRole.Name);
UserInfo.getUserId() gets the current logged-in user’s ID.
The query retrieves Profile Name and User Role Name for that user.
3. Important Notes
Every user must have a Profile, but UserRole can be null.
If you only need the profile name, use:
System.debug(UserInfo.getProfileId());
However, this returns the Profile ID, not the name, so a query is needed.
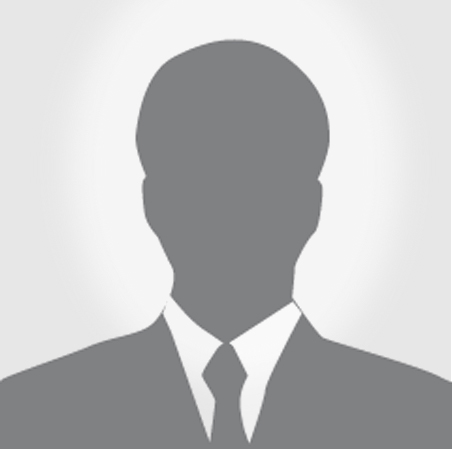

Use the SELECT clause in combination with Relationship Queries in SOQL to retrieve the fall guys names of Roles (UserRole.Name) and Profiles (Profile.Name) directly from the User table.SELECT Id, UserRoleId, ProfileId, UserRole.Name, Profile.NameSELECT Id, UserRoleId, ProfileId, UserRole.Name, Profile.Name
SELECT Id, UserRoleId, ProfileId, UserRole.Name, Profile.Name
FROM User
WHERE Id = 'USER_ID_HERE'
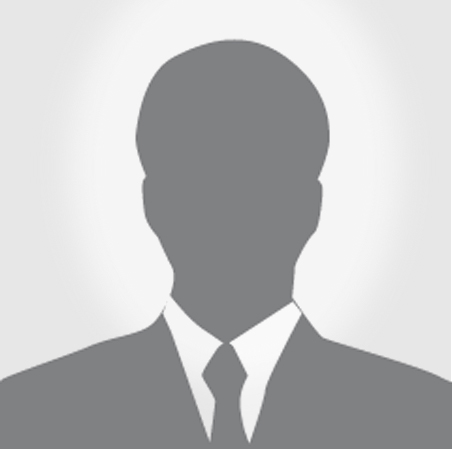

To retrieve the profile name and role name from a user object, you typically need to access specific fields related to the user in your database or application. The exact method depends on the context (e.g., a Salesforce environment, a custom application with a database, etc.). Below, I'll provide examples for both Salesforce and a generic database application.
In Salesforce
In Salesforce, each user is associated with a profile and a role. You can retrieve the profile name and role name using SOQL (Salesforce Object Query Language).
Example SOQL Query
SELECT Id, Name, Profile.Name, UserRole.Name FROM User WHERE Id = 'someUserId'
Explanation
- Id: The unique identifier for the user.
- Name: The name of the user.
- Profile.Name: The name of the profile associated with the user.
- UserRole.Name: The name of the role associated with the user.
In a Custom Application with a Database
If you have a custom application with a relational database, you typically have tables like users, profiles, and roles. You can use SQL to join these tables and get the desired information.
Example SQL Query
SELECT users.id, users.name, profiles.name AS profile_name, roles.name AS role_name
FROM users
JOIN profiles ON users.profile_id = profiles.id
JOIN roles ON users.role_id = roles.id
WHERE users.id = 'someUserId';
- Explanation
- users.id: The unique identifier for the user.
- users.name: The name of the user.
- profiles.name: The name of the profile associated with the user.
- roles.name: The name of the role associated with the user.
- In a Programming Context
If you're working within a specific programming context (e.g., a Python application using an ORM), the approach will vary. Here’s an example using Python with SQLAlchemy:
Python with SQLAlchemy Example
from sqlalchemy.orm import sessionmaker
from your_model_definitions import User, Profile, Role
# Assuming you have a configured engine
Session = sessionmaker(bind=engine)
session = Session()
user_id = 'someUserId'
user = session.query(User).filter(User.id == user_id).first()
if user:
profile_name = user.profile.name
role_name = user.role.name
print(f"Profile Name: {profile_name}, Role Name: {role_name}")
else:
print("User not found")
Explanation
This code snippet uses SQLAlchemy to query a user and get the related profile and role names.
User, Profile, and Role are ORM models.
The relationships between User, Profile, and Role should be properly defined in your ORM models.
Conclusion
The exact method to retrieve the profile name and role name from a user object depends on the environment and tools you're using. Whether it's Salesforce with SOQL, a relational database with SQL, or an ORM in a programming language, the general approach involves querying the user and their associated profile and role.