
How to work with httpresponse apex?
I am able to do a successful callout and get the response just fine. Having issues referencing the data inside the response.getBody(). What method do you use to be able to reference the data sent back in the body?
Response body is saying: {"success":true,"data":{"account_number":56789}}
How do I grab the Account Number to reference in the controller?
So, the httpresponse apex you are receiving is a JSON string. You can parse it like this:
String json = response.getBody();
Map responseMap = (Map) JSON.deserializeUntyped(json);
Then, you can access it like this:
Map dataMap = (Map)responseMap.get('data');
String accountNumber = (String)dataMap.get('account_number');
Your Answer
Answers (2)
If you're working with HttpResponse in Apex and want to handle API responses effectively, here’s what you need to know!
1. What is HttpResponse in Apex?
- HttpResponse is part of Salesforce’s Apex HTTP callout framework.
- It is used to capture responses from external APIs when making HTTP requests.
2. How to Use HttpResponse in Apex?
- To make an API request and handle the response, follow these steps:
- Basic Example: Sending a GET Request
public class CalloutExample {
public static void makeHttpCallout() {
Http http = new Http();
HttpRequest request = new HttpRequest();
request.setEndpoint('https://jsonplaceholder.typicode.com/posts/1');
request.setMethod('GET');
HttpResponse response = http.send(request);
if (response.getStatusCode() == 200) {
System.debug('Response Body: ' + response.getBody());
} else {
System.debug('Error: ' + response.getStatus());
}
}
}
getBody() → Retrieves the response content as a string.
getStatusCode() → Returns the HTTP status code (e.g., 200 for success).
getStatus() → Returns the status message (e.g., "OK", "Not Found").
3. Handling JSON Responses
If the API returns JSON, parse it using JSON.deserialize():
public class Post {
public Integer id;
public String title;
}
HttpResponse response = http.send(request);
if (response.getStatusCode() == 200) {
Post post = (Post) JSON.deserialize(response.getBody(), Post.class);
System.debug('Post Title: ' + post.title);
}
4. Final Thoughts
- Use getBody() to extract API data.
- Always check the status code before processing responses.
- Use JSON.deserialize() to parse JSON responses easily.
1 Month
In Salesforce, Apex is used to write custom server-side logic. When dealing with HTTP responses in Apex, you typically interact with the HttpResponse class. This class is part of the Http namespace and allows you to handle responses from HTTP requests. Here is a guide on how to work with HttpResponse in Apex:
Step-by-Step Guide
Create an HTTP Request:
Use the HttpRequest class to set up your HTTP request.
Send the HTTP Request:
Use the Http class to send the request and receive the response.
Handle the HTTP Response:
Use the HttpResponse class to process the response from the server.
Example Code
Below is an example of making a simple GET request to a REST API and handling the response:
// Import necessary classesHttp http = new Http();HttpRequest request = new HttpRequest();HttpResponse response;// Set up the requestrequest.setEndpoint('https://api.example.com/data');request.setMethod('GET');// Optionally, you can set headersrequest.setHeader('Content-Type', 'application/json');request.setHeader('Authorization', 'Bearer your_access_token');try { // Send the request response = http.send(request); // Handle the response if (response.getStatusCode() == 200) { // Success String responseBody = response.getBody(); // Parse the response if needed // For example, if the response is JSON: Map jsonResponse = (Map) JSON.deserializeUntyped(responseBody); System.debug('Response: ' + jsonResponse); } else { // Handle non-200 status codes System.debug('Failed with status code: ' + response.getStatusCode()); System.debug('Response: ' + response.getBody()); }} catch (Exception e) { // Handle any exceptions System.debug('Exception: ' + e.getMessage());}
Detailed Explanation
Set Up the Request:
- Create an HttpRequest object.
- Set the endpoint URL and HTTP method (e.g., GET, POST).
- Optionally, set headers (e.g., Content-Type, Authorization).
Send the Request:
Use the Http class's send method to send the request and receive an HttpResponse object.
Process the Response:
- Check the status code using response.getStatusCode().
- If the status code is 200 (OK), retrieve the response body using response.getBody().
- Optionally, parse the response body. If it's JSON, you can use JSON.deserializeUntyped to convert it to a map.
Handle Errors and Exceptions:
- If the status code is not 200, handle the error accordingly.
- Use a try-catch block to handle exceptions that may occur during the request.
Common Methods in HttpResponse
- getBody(): Returns the response body as a string.
- getStatusCode(): Returns the status code of the HTTP response.
- getStatus(): Returns the status line returned by the HTTP response.
- getHeader(String key): Returns the value of the specified header from the response.
Example for a POST Request
Here is an example for making a POST request:
Http http = new Http();HttpRequest request = new HttpRequest();HttpResponse response;request.setEndpoint('https://api.example.com/data');request.setMethod('POST');request.setHeader('Content-Type', 'application/json');request.setHeader('Authorization', 'Bearer your_access_token');// Set the request bodyString requestBody = '{"key1":"value1", "key2":"value2"}';request.setBody(requestBody);try { response = http.send(request); if (response.getStatusCode() == 201) { String responseBody = response.getBody(); Map jsonResponse = (Map) JSON.deserializeUntyped(responseBody); System.debug('Response: ' + jsonResponse); } else { System.debug('Failed with status code: ' + response.getStatusCode()); System.debug('Response: ' + response.getBody()); }} catch (Exception e) { System.debug('Exception: ' + e.getMessage());}
By following these steps, you can effectively handle HTTP responses in Apex, making it possible to integrate with external web services and APIs.
10 Months
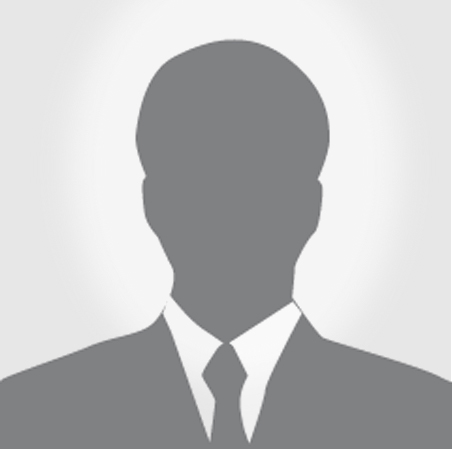

If you're working with HttpResponse in Apex and want to handle API responses effectively, here’s what you need to know!
1. What is HttpResponse in Apex?
- HttpResponse is part of Salesforce’s Apex HTTP callout framework.
- It is used to capture responses from external APIs when making HTTP requests.
2. How to Use HttpResponse in Apex?
- To make an API request and handle the response, follow these steps:
- Basic Example: Sending a GET Request
public class CalloutExample {
public static void makeHttpCallout() {
Http http = new Http();
HttpRequest request = new HttpRequest();
request.setEndpoint('https://jsonplaceholder.typicode.com/posts/1');
request.setMethod('GET');
HttpResponse response = http.send(request);
if (response.getStatusCode() == 200) {
System.debug('Response Body: ' + response.getBody());
} else {
System.debug('Error: ' + response.getStatus());
}
}
}
getBody() → Retrieves the response content as a string.
getStatusCode() → Returns the HTTP status code (e.g., 200 for success).
getStatus() → Returns the status message (e.g., "OK", "Not Found").
3. Handling JSON Responses
If the API returns JSON, parse it using JSON.deserialize():
public class Post {
public Integer id;
public String title;
}
HttpResponse response = http.send(request);
if (response.getStatusCode() == 200) {
Post post = (Post) JSON.deserialize(response.getBody(), Post.class);
System.debug('Post Title: ' + post.title);
}
4. Final Thoughts
- Use getBody() to extract API data.
- Always check the status code before processing responses.
- Use JSON.deserialize() to parse JSON responses easily.
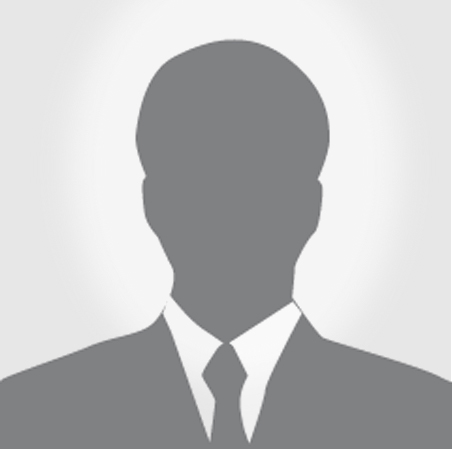

In Salesforce, Apex is used to write custom server-side logic. When dealing with HTTP responses in Apex, you typically interact with the HttpResponse class. This class is part of the Http namespace and allows you to handle responses from HTTP requests. Here is a guide on how to work with HttpResponse in Apex:
Step-by-Step Guide
Create an HTTP Request:
Use the HttpRequest class to set up your HTTP request.
Send the HTTP Request:
Use the Http class to send the request and receive the response.
Handle the HTTP Response:
Use the HttpResponse class to process the response from the server.
Example Code
Below is an example of making a simple GET request to a REST API and handling the response:
// Import necessary classesHttp http = new Http();HttpRequest request = new HttpRequest();HttpResponse response;// Set up the requestrequest.setEndpoint('https://api.example.com/data');request.setMethod('GET');// Optionally, you can set headersrequest.setHeader('Content-Type', 'application/json');request.setHeader('Authorization', 'Bearer your_access_token');try { // Send the request response = http.send(request); // Handle the response if (response.getStatusCode() == 200) { // Success String responseBody = response.getBody(); // Parse the response if needed // For example, if the response is JSON: Map jsonResponse = (Map) JSON.deserializeUntyped(responseBody); System.debug('Response: ' + jsonResponse); } else { // Handle non-200 status codes System.debug('Failed with status code: ' + response.getStatusCode()); System.debug('Response: ' + response.getBody()); }} catch (Exception e) { // Handle any exceptions System.debug('Exception: ' + e.getMessage());}
Detailed Explanation
Set Up the Request:
- Create an HttpRequest object.
- Set the endpoint URL and HTTP method (e.g., GET, POST).
- Optionally, set headers (e.g., Content-Type, Authorization).
Send the Request:
Use the Http class's send method to send the request and receive an HttpResponse object.
Process the Response:
- Check the status code using response.getStatusCode().
- If the status code is 200 (OK), retrieve the response body using response.getBody().
- Optionally, parse the response body. If it's JSON, you can use JSON.deserializeUntyped to convert it to a map.
Handle Errors and Exceptions:
- If the status code is not 200, handle the error accordingly.
- Use a try-catch block to handle exceptions that may occur during the request.
Common Methods in HttpResponse
- getBody(): Returns the response body as a string.
- getStatusCode(): Returns the status code of the HTTP response.
- getStatus(): Returns the status line returned by the HTTP response.
- getHeader(String key): Returns the value of the specified header from the response.
Example for a POST Request
Here is an example for making a POST request:
Http http = new Http();HttpRequest request = new HttpRequest();HttpResponse response;request.setEndpoint('https://api.example.com/data');request.setMethod('POST');request.setHeader('Content-Type', 'application/json');request.setHeader('Authorization', 'Bearer your_access_token');// Set the request bodyString requestBody = '{"key1":"value1", "key2":"value2"}';request.setBody(requestBody);try { response = http.send(request); if (response.getStatusCode() == 201) { String responseBody = response.getBody(); Map jsonResponse = (Map) JSON.deserializeUntyped(responseBody); System.debug('Response: ' + jsonResponse); } else { System.debug('Failed with status code: ' + response.getStatusCode()); System.debug('Response: ' + response.getBody()); }} catch (Exception e) { System.debug('Exception: ' + e.getMessage());}
By following these steps, you can effectively handle HTTP responses in Apex, making it possible to integrate with external web services and APIs.